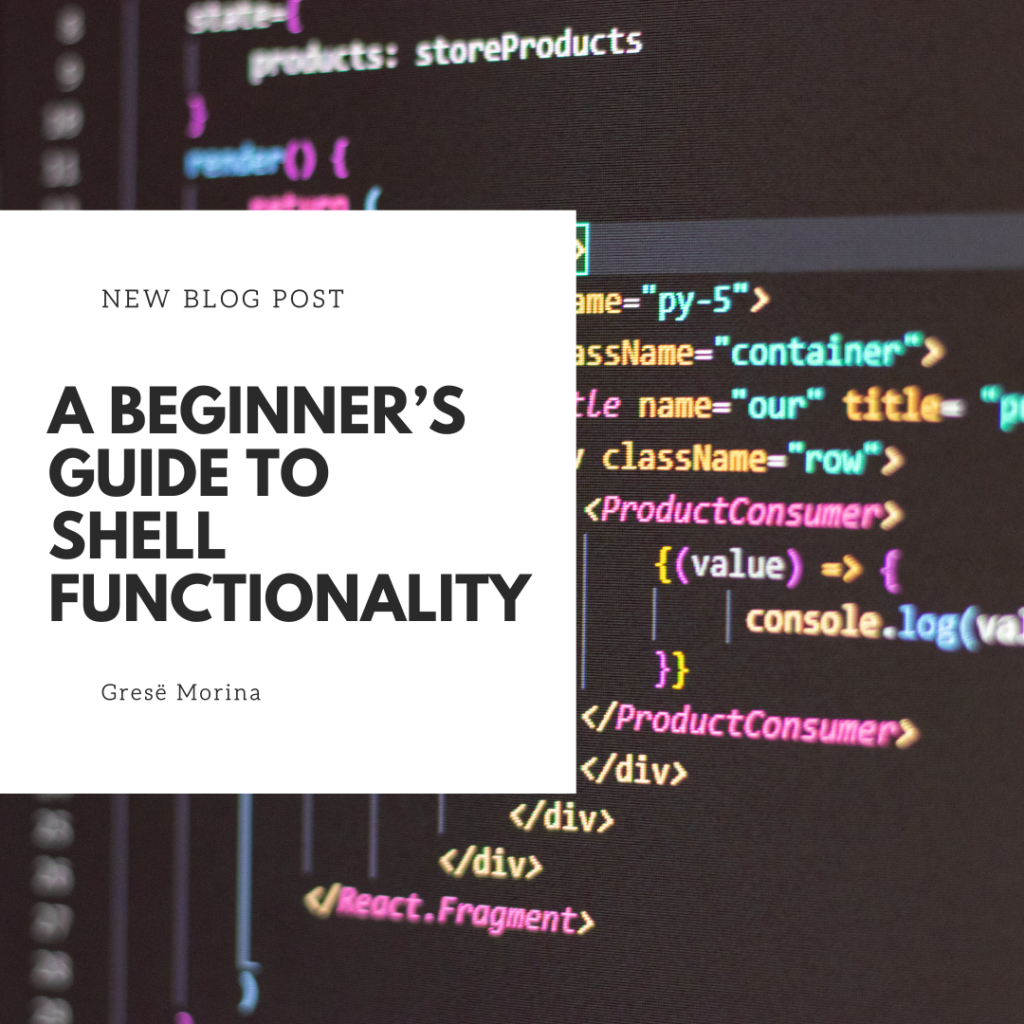
As I embark on the journey of writing a shell in C, I’ve come to realize that writing a shell in C involves understanding several key functions and features that make it effective. Here are some key functions that a shell must perform. While I’m sure there are some critical aspects I might have overlooked, this list captures the essentials:
Command Parsing: The shell must interpret the input provided by the user to distinguish between commands and their corresponding arguments. For example, in the command
ls -la LICENSE
, the shell identifiesls
as the command and-la LICENSE
as the arguments.Command Expansion: The shell needs to expand wildcard characters. For instance,
ls *
should be translated intols file1 file2 file3 ...
, where it lists all files in the current directory.Pipes and Redirection: When executing commands like
ls | grep blah
, the shell must facilitate the piping of output from one command (ls
) into another (grep
). Additionally, it must handle redirection, allowing output to be sent to files or other processes.Signal Handling: The shell should manage signals sent to processes. For example, pressing
Ctrl+C
sends an interrupt signal to the currently running process. Although I am still working to fully understand this aspect, it is a crucial part of process management.Process Management: The shell must support job control, allowing users to background and foreground processes using commands like
Ctrl+Z
,fg
, andbg
.Shell Scripting: The shell should enable users to create scripts, including control structures such as loops and conditionals.
Additional Considerations
One important function that a shell does not handle directly is determining which command to execute using the $PATH
environment variable. This task is typically managed by the exec
family of functions.