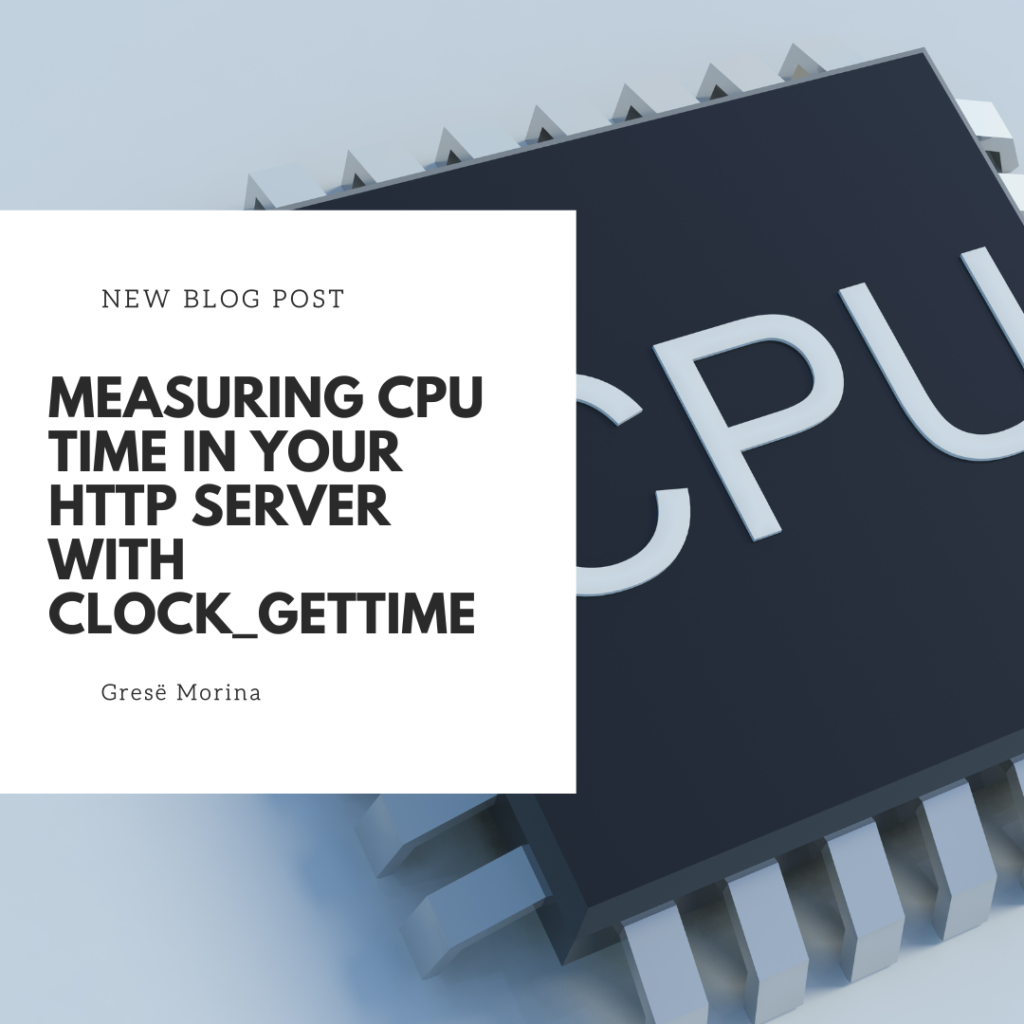
When optimizing a slow program, the first thing to assess is whether it’s spending time processing calculations on the CPU or waiting for other resources, like disk or network I/O.
Recently, I learned that some colleagues were measuring how much CPU time each HTTP request takes in their server. Curious about how they achieved this, I found out that the Linux kernel provides a straightforward way to track CPU usage: the clock_gettime
function.
What is clock_gettime?
clock_gettime
is a system call (and corresponding C library function) that does more than just give you the current time. It provides high-resolution timing, which is invaluable for performance profiling.
Here are some key flags you can use with clock_gettime
, as outlined in the man
page:
- CLOCK_REALTIME: This is the system-wide real-time clock. Modifying it requires specific privileges.
- CLOCK_MONOTONIC: This clock measures time from an unspecified starting point and cannot be adjusted. It’s useful for measuring elapsed time.
- CLOCK_MONOTONIC_RAW: Similar to
CLOCK_MONOTONIC
, but it offers raw hardware time unaffected by adjustments (like NTP). - CLOCK_PROCESS_CPUTIME_ID: This flag gives you a high-resolution timer for the CPU time consumed by the current process.
- CLOCK_THREAD_CPUTIME_ID: This provides CPU time specific to the current thread.
How to Measure CPU Time for HTTP Requests
To measure how much CPU time each HTTP request takes, you can use the CLOCK_PROCESS_CPUTIME_ID
flag. Here’s a simple example of how it works:
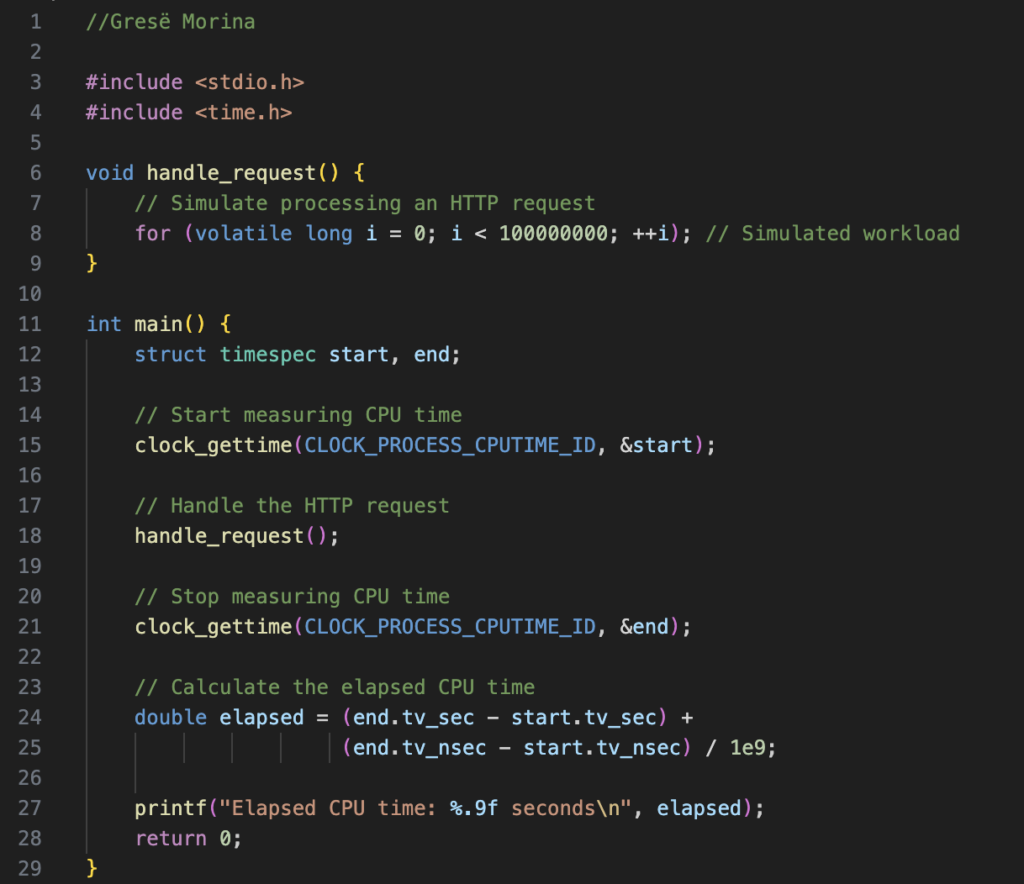
Using clock_gettime
with the CLOCK_PROCESS_CPUTIME_ID
flag allows you to precisely measure the CPU time taken by your application, which is essential for performance tuning. This way, you can ensure that your HTTP server processes requests efficiently and can pinpoint any performance bottlenecks effectively.
Feel free to adapt this code and approach in your own projects! Happy coding!